How can I do spot trading with the Jupyter Notebook?
Learn how you can do simple Spot trading by calling the functions in the python-okx library on a Jupyter Notebook.
1. How can I run Python code snippets on a Jupyter Notebook?
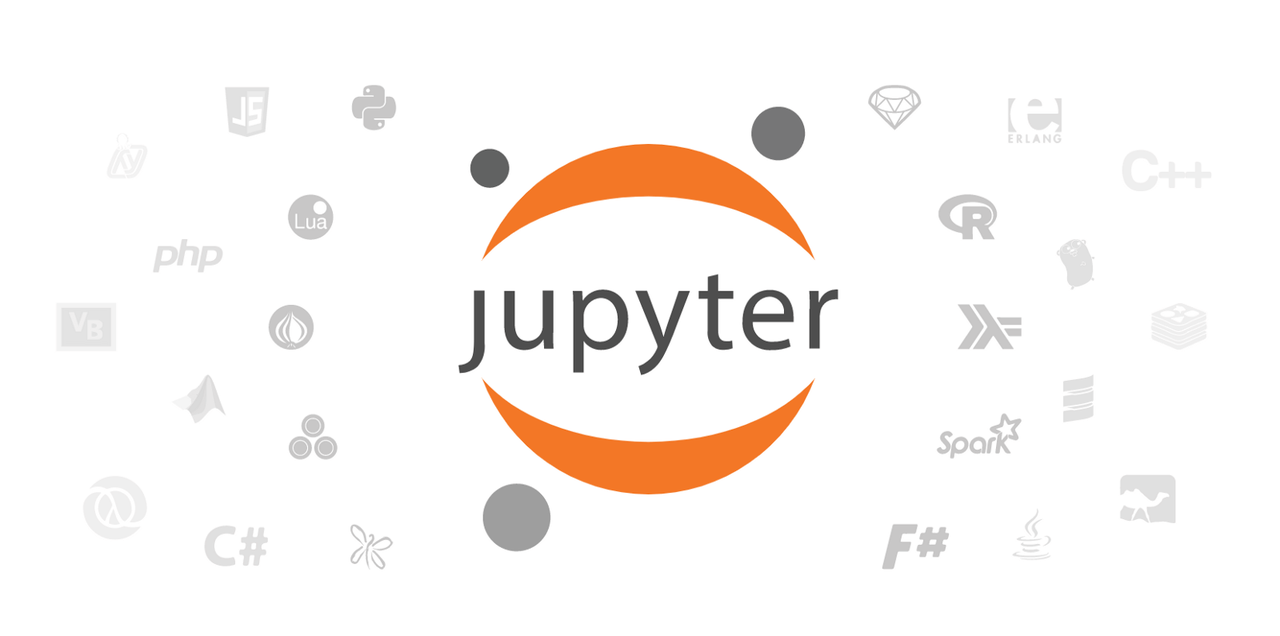
The Jupyter Notebook is an incredibly powerful and easy-to-use tool for Python development and data analysis. You can run a Jupyter Notebook server on Windows, Mac OS or Linux. This tutorial provides a pretty comprehensive guide on how to get a Jupyter Notebook up and running.
2. How can I install the python-okx package?
Once you start running a Jupyter Notebook, you can install the python-okx package by simply running the pip install python-okx
in the notebook or in a terminal (or via command prompt for Windows):

3. How can I create API keys?
After signing in to our platform, go to Trade > Demo trading to create API keys for testing purposes
Open Demo trading page
Go to Profile and select Demo Trading API to create API keys.
Open API to create API keys
Create API keys for the different master/sub accounts you might have
Select Create API key
Select Trade in the Permissions to trade with the API key
Select Trade in Permissions
Now you have access to your API key, your Secret key, and your passphrase. Keep them in a safe place!
Instantiate python variables to save your API details in the notebook for later usage
Python1 api_key = "xxxxx" 2 secret_key = "xxxxx" 3 passphrase = "xxxxxx"
4. How can I import OKX modules?
In python-okx, there are several modules based on our REST API modules. Read our guide to learn how to import OKX modules.
Trade
BlockTrading
Funding
Account
Convert
Earning
SubAccount
MarketData
PublicData
TradingData
Status
NDBroker
FDBroker
To import the Trade module, you can run:
1 import okx.Trade as Trade
Now you are ready to use the comprehensive features available in python-okx!
5. How can I access our market data?
Learn more on how to access our market data and refer to the code below.
1 import okx.MarketData as MarketData
2 flag = "1" # live trading: 0, demo trading: 1
3 marketDataAPI = MarketData.MarketAPI(flag=flag)
4 result = marketDataAPI.get_tickers(instType="SPOT")print(result)
6. How can I read the available trading pairs?
Learn more on how to read our available trading pairs and refer to the code below.
1 import okx.Account as Account
2
3 # API initialization
4 apikey = "YOUR_API_KEY"
5 secretkey = "YOUR_SECRET_KEY"
6 passphrase = "YOUR_PASSPHRASE"
7
8 flag = "1" # Production trading: 0, Demo trading: 1
9
10 accountAPI = Account.AccountAPI(apikey, secretkey, passphrase, False, flag)
11
12 result = accountAPI.get_instruments(instType="SPOT")
13 print(result)
7. How can I read my account balance?
For more information on how to read your account balance, please read our dedicated guide.
Note: for spot trading under "cash" tdMode
, you mainly need to check the cashBal
, frozenBal
parameters for each ccy
under details
, and the totalEq
parameter.
1 import okx.Account as Account
2 flag = "1" # live trading: 0, demo trading: 1
3
4 accountAPI = Account.AccountAPI(api_key, secret_key, passphrase, False, flag)
5
6 result = accountAPI.get_account_balance()
7 print(result)
8. How can I access the four different account modes?
In our unified account system, there are four account modes:
Spot mode
Spot and futures mode
Multi-currency margin mode
Portfolio margin mode
To understand the difference between different account modes and how to set up the account mode via the web UI, please read our dedicated guide.
In margin mode or trade mode, the parameter tdMode
determines how your position is going to be margined, which you need to set every time you place a new order.
For spot trading under spot or spot and futures mode, please set tdMode
='cash'.
For spot trading under multi-currency margin or portfolio margin mode, please set tdMode
= 'cross'.
You'll find below a quick explanation of how to figure out what mode your current account is configured as.
9. How can I figure out what mode my current account configured as?
For more information on how to figure out what mode your current account is configured as, please read our dedicated guide and enter the acctLv
parameter.
1 import okx.Account as Account
2
3 flag = "1" # live trading: 0, demo trading: 1
4
5 accountAPI = Account.AccountAPI(api_key, secret_key, passphrase, False, flag)
6 result = accountAPI.get_account_config()
7 print(result)
8
9 if result['code'] == "0":
10 acctLv = result["data"][0]["acctLv"]
11 if acctLv == "1":
12 print("Simple mode")
13 elif acctLv == "2":
14 print("Single-currency margin mode")
15 elif acctLv == "3":
16 print("Multi-currency margin mode")
17 elif acctLv == "4":
18 print("Portfolio margin mode")
10. How can I place spot orders under Spot / Spot and futures mode?
10.1 How can I place a limit order?
For more information on how to place a limit order under a spot or spot and futures mode, please read our dedicated guide.
Here's an example of buying 0.01 BTC at the price of 19000 USDT.
1 # limit order
2 result = tradeAPI.place_order(
3 instId="BTC-USDT",
4 tdMode="cash",
5 side="buy",
6 ordType="limit",
7 px="19000",
8 sz="0.01"
9 )
10 print(result)
11
12 if result["code"] == "0":
13 print("Successful order request,order_id = ",result["data"][0]["ordId"])
14 else:
15 print("Unsuccessful order request,error_code = ",result["data"][0]["sCode"], ", Error_message = ", result["data"][0]["sMsg"])
10.2 How can I place a market order?
For more information on how to place a market order under a spot or spot and futures mode, please read our dedicated guide.
Here's an example of buying BTC that is worth 100 USD at the current market price.
1 # market order
2 result = tradeAPI.place_order(
3 instId="BTC-USDT",
4 tdMode="cash",
5 side="buy",
6 ordType="market",
7 sz="100",
8 )
9 print(result)
10.3 How can I use the target currency parameter tgtCcy while spot trading?
In spot trading, the parameter tgtCcy
determines the unit of the size parameter sz
, which can be either the base currency or the quote currency of the trading pair. For example, in the pair BTC-USDT, the base currency is BTC and the quote currency is USDT.
By default, tgtCcy
= quote_ccy for buy orders, which means the sz
you specified is in terms of the quote currency. Meanwhile, the default value of tgtCcy
for sell orders is base_ccy, which means the sz
you specified is in terms of the base currency.
In the example below, you are about to place a market order to buy BTC that is worth 100 USD.
1 # market order
2 result = tradeAPI.place_order(
3 instId="BTC-USDT",
4 tdMode="cash",
5 side="buy",
6 ordType="market",
7 sz="100",
8 tgtCcy="quote_ccy" # this determines the unit of the sz parameter.
9 )
10 print(result)
10.4 How can I use the client order ID parameter clOrdId?
When you place an order, you can specify your own client order ID by specifying the parameter clOrdId
, which can later be used as an identifier in place of ordId
when calling an order cancellation, amendment or retrieval endpoint.
1 # market order
2 result = tradeAPI.place_order(
3 instId="BTC-USDT",
4 tdMode="cash",
5 side="buy",
6 ordType="market",
7 sz="100",
8 clOrdId="003" # you can define your own client defined order ID
9 )
10 print(result)
11. How can I get details about a certain order?
For more information on how to get details about a certain order, please read our dedicated guide.
11.1 Using ordId
1 result = tradeAPI.get_order(instId="BTC-USDT", ordId="497819823594909696")
2 print(result)
11.2 Using clOrdId
1 result = tradeAPI.get_order(instId="BTC-USDT", clOrdId="002")
2 print(result)
12. How can I cancel an order?
For more information on how to cancel an order, please read our dedicated guide.
You can also use clOrdId
instead of ordId
.
1 result = tradeAPI.cancel_order(instId="BTC-USDT", ordId = "489093931993509888")
2 print(result)
13. How can I amend an order?
For more information on how to amend an order, please read our dedicated guide.
You can also use clOrdId
instead of ordId
.
1 result = tradeAPI.amend_order(
2 instId="BTC-USDT",
3 ordId="489103565508685824",
4 newSz="0.012"
5 )
6 print(result)
14. How can I access the list of open orders?
For more information on how to access the list of open orders, please read our dedicated guide.
1 result = tradeAPI.get_order_list()
2 print(result)
15. How can I access order history?
15.1 For the last 7 days
For more information on how to access the order history for the last 7 days, please read our dedicated guide.
1 result = tradeAPI.get_orders_history(
2 instType="SPOT"
3 )
4 print(result)
15.2 For the last 3 months
For more information on how to access the order history for the last 3 months, please read our dedicated guide.
1 result = tradeAPI.get_orders_history_archive(
2 instType="SPOT"
3 )
4 print(result)
16. How can I go further with the OKX API with a Jupyter Notebook?
For more examples, download the full Jupyter Notebook here.
If you have any questions about our APIs, you can join our API community and raise them in the community.